Before Start
While defining a constant in a project I was examining, I saw that iota was written in the value section and I was like a rabbit with a light shined in its eye. I immediately typed Google -> what is iota golang? and I am writing this article because I could not find a Turkish source. Oh, and since cryptocurrencies are extremely popular nowadays, I came across IOTA as a coin. Most of the time, the values of variables are important to us, but what if these values don't matter, it is enough to just exist? At this point, iota comes into play.
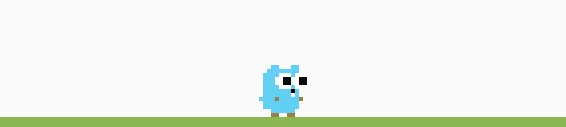
In the context of Go programming language, IOTA is a keyword used within constant declarations to simplify definitions of incrementing numbers. When you use iota in a constant declaration, it is automatically initialized to zero and then incremented by 1 for each subsequent constant specification in the declaration.
const (
kitapKategorisi = 0
sağlıkKategorisi = 1
giyimKategorisi = 2
)
At this point, our only goal is that the variables exist and have different values. If we do this using IOTA, iota increments in the form of 0-2-3-4....:
const (
kolay = 0
orta = 1
zor = 2
)
fmt.Printf("kolay: %d\norta: %d\nzor: %d\n", kolay, orta, zor)
The output will look like this:
kolay: 0
orta : 1
zor : 2
Operations with IOTA
package main
import "fmt"const (
_ = iota
a = iota + 10 // her seferinde iota yazmaya gerek yok.
b int = iota * 10
c float64 = iota + 5.1
)
func main() {
fmt.Printf("%v — %T\n", a, a)
fmt.Printf("%v — %T\n", b, b)
fmt.Printf("%v — %T\n", c, c)
}
Output=>
11 — int
20 — int
8.1 — float64
Resetting IOTA's Increase
IOTA is reset when we open a new const definition field.
package main
import "fmt"
const (
a = iota
b int = iota
)
const (
c = iota
)
func main() {
fmt.Printf("%v — %T\n", a, a)
fmt.Printf("%v — %T\n", b, b)
fmt.Printf("%v — %T\n", c, c)
}
Output=>
0 — int
1 — int
0 — int
Creating Bitmask with IOTA
package main
import "fmt"
const (
Secure = 1 << iota // 0b001
Authn // 0b010
Ready // 0b100
)
func main() {
ConnState := Secure | Authn
fmt.Printf(` Secure: 0x%x (0b%03b)
Authn: 0x%x (0b%03b)
ConnState: 0x%x (0b%03b)
`, Secure, Secure, Authn, Authn, ConnState, ConnState)
}
Refrences